Muy buen día.
Hoy les comparto un pequeño programa que te permite
obtener todos tus archivos con extensión .cs de una carpeta determinada.
lo que tienes que realizar, es crear un proyecto de WPF, y en el MainWindow, tienes que remplazar el codigo tanto del code behind asi como del xaml.
aqui tenemos el xaml que tienes que pegar en tu pantalla MainWindow
<Window x:Class="viwer.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="File
Viewer" Height="350" Width="525">
<Grid>
<Button Content="Show .cs files" Height="23" HorizontalAlignment="Right" Margin="0,24,12,0" Name="btnGetFiles" VerticalAlignment="Top" Width="114" Click="btnGetFiles_Click" />
<TextBox Height="23" HorizontalAlignment="Left" Margin="12,24,0,0" Name="txtPath" VerticalAlignment="Top" Width="359" />
<TextBox Height="246" HorizontalAlignment="Left" Margin="12,53,0,0" Name="TxtFilesBox" VerticalAlignment="Top" Width="479" />
</Grid>
</Window>
Y aquí tenemos el code-behind:
using
System;
using
System.Windows;
using
System.Collections.Generic;
using
System.IO;
using
System.Linq;
using
System.Windows.Documents;
namespace
viwer
{
public partial class MainWindow : Window
{
public
MainWindow()
{
InitializeComponent();
}
private void
btnGetFiles_Click(object sender, RoutedEventArgs e)
{
try
{
string
path = txtPath.Text;
string
files = String.Empty;
string[]
listOfFiles = Directory.GetFiles(path);
List<string> listOfNameOfFiles = new List<string>();
foreach
(var file in
listOfFiles)
{
FileInfo
infoFile = new FileInfo(file);
if
(infoFile.Name.Contains(".cs"))
listOfNameOfFiles.Add(infoFile.Name.Split('.')[0]);
}
foreach
(string file in
listOfNameOfFiles)
files += file + "\n";
TxtFilesBox.Text = files;
}
catch
{
MessageBox.Show("Error to try to get the files of the current
path");
}
}
}
}
Analicemos un poco la siguiente clase invocando a su método estático.
Directory.GetFiles(path)
La clase Directory nos permite utilizar muchas
funcionalidades útiles a la hora de manipular directorios de Windows, en este
caso, estamos haciendo uso del método estático GetFiles(string path).
Con este método obtendremos todos los archivos con su
ruta completa.
Para poder obtener solamente el nombre del archivo,
tenemos que hacer uso de otra clase llamada:
FileInfo
Esta clase tiene una propiedad con nombre Name, es
la que nosotros utilizaremos para consultar nuestros archivos “.cs”
Veamos como se ve en ejecución.
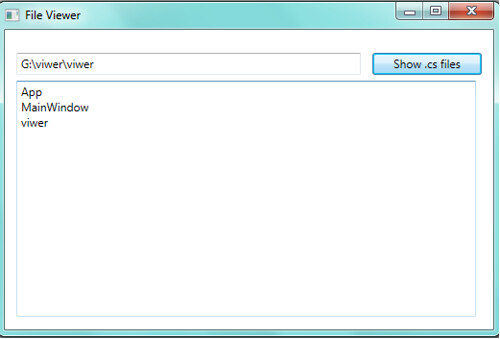